Stream Back-Pressure and Cancellation with Vercel AI SDK
Let's learn about two important concepts in the Vercel AI SDK: Stream Back-pressure and cancellation.
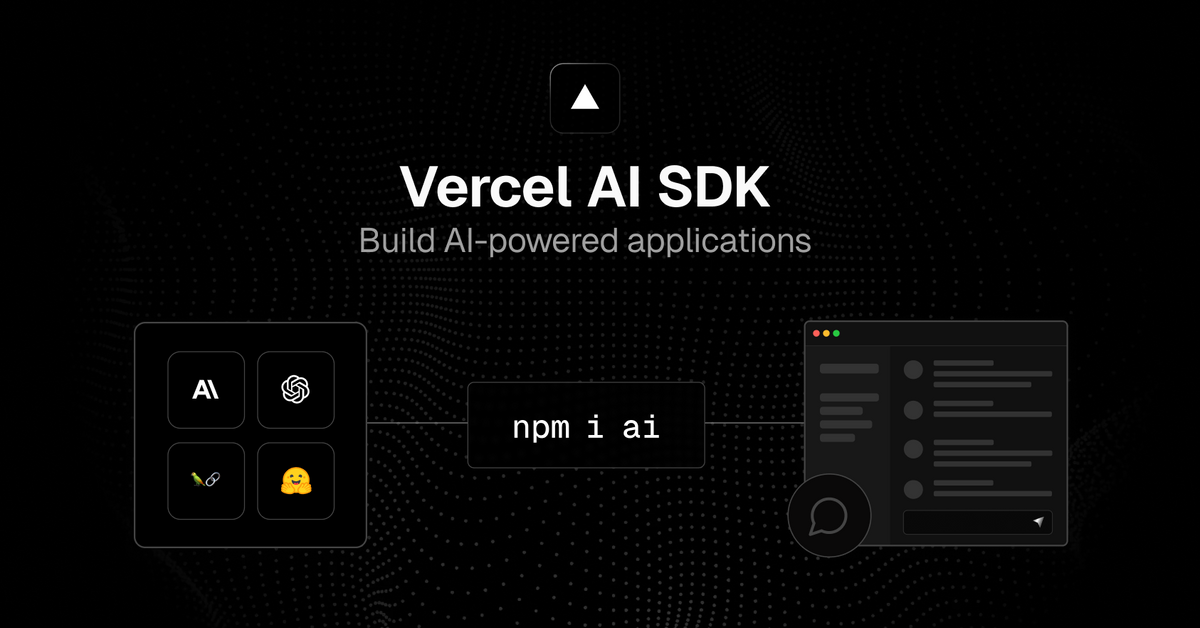
Understanding the principles of back-pressure and cancellation when working with streams is vital for efficient data processing, especially in the context of AI integrations. This post dives deep into these principles using Vercel AI SDK, providing insights that can be crucial for advanced users.
Subscribe or follow me on Twitter for more content like this!
Introduction
This page focuses on understanding back pressure and cancellation when working with streams. You do not need to know this information to use the Vercel AI SDK, but for those interested, it offers a deeper dive into why and how the SDK optimally streams responses.
Key Ideas
- Understanding Back-pressure and Cancellation: These concepts are useful for handling streams, especially in AI contexts, ensuring efficient data processing.
- Eager vs. Lazy Approach: The eager approach leads to an ever-expanding queue of items, while the lazy approach respects back-pressure, pairing the yield and read actions, thus optimizing data handling.
- Using a Pull Handler for Back-pressure: The pull handler ensures that data is produced only when demanded by the consumer, preventing unnecessary memory usage.
- Cancellation through Manual Iteration: Proper cancellation handling ensures that once reads stop, the yields will also stop, conserving resources and preventing continuous generation of unnecessary data.
- Integration with AI Responses: A proper handling of back-pressure and cancellation ensures optimal performance when working with AI services like AIBot, allowing efficient garbage collection and freeing connections.
- Real-world Impact: The application of these principles leads to robust systems, capable of handling infinite streams without overwhelming resources, essential for performance and responsiveness in applications integrating AI services.
These key ideas provide a roadmap to managing data streams effectively, not just in the context of Vercel AI SDK, but in any context where streams of data are used, and efficiency and responsiveness are critical.
Understanding Back-Pressure and Cancellation
Back-Pressure
Back-pressure refers to the control mechanism where the rate of data production matches the rate of consumption. It prevents the accumulation of excess data in a system, thus preventing potential bottlenecks and crashes.
Cancellation
Cancellation refers to the ability to interrupt and halt an ongoing operation or task. This can prevent unnecessary processing and consumption of resources.
When to Consider Back-Pressure and Cancellation
Handling Large or Infinite Data Streams
In situations where data streams are large or infinite, and there is a significant difference between the rates of production and consumption, back-pressure is vital. Without it, an uncontrolled accumulation of data might lead to system failure.
Resource-Intensive Applications
In applications where resources are precious and should be carefully managed, the cancellation of unnecessary tasks becomes a necessity. It helps to conserve resources and promotes efficiency in the system.
Real-Time Processing
For real-time systems where data flow needs to be perfectly synchronized, both back-pressure and cancellation mechanisms are vital. A delay or lag can lead to incorrect processing or failure of the system.
When Back-Pressure and Cancellation Might Be Overkill
Small, Controlled Data Streams
In systems where data streams are small, predictable, and the difference between production and consumption rates is negligible, implementing back-pressure might be over-complication. The overhead of implementing and managing it might outweigh the benefits.
Low Priority or Non-Critical Operations
In tasks or operations where resources are not a constraint, and the accuracy or timing is not critical, the need for cancellation might be unnecessary. Here, the time and effort required to implement cancellation may outweigh the minimal benefits gained.
Limited Resource Investment
In cases where the system or application is not consuming significant resources, neither back-pressure nor cancellation might be required. The overhead of implementing these controls may lead to more complexity without significant gains.
Back-pressure and Cancellation with Streams: An Example
Let's set the stage by introducing a simple example program, based on the Vercel AI SDK docs:
// Generate positive integers
async function* integers() {
let i = 1;
while (true) {
yield i++;
await sleep(100);
}
}
// Delay function
function sleep(ms) {
return new Promise((resolve) => setTimeout(resolve, ms));
}
// Create a ReadableStream from a generator
function createStream(iterator) {
return new ReadableStream({
async start(controller) {
for await (const v of iterator) {
controller.enqueue(v);
}
controller.close();
},
});
}
// Function to collect data from the stream
async function run() {
const stream = createStream(integers());
const reader = stream.getReader();
for (let i = 0; i < 10000; i++) {
const { value } = await reader.read();
console.log(`read ${value}`);
await sleep(1000);
}
}
run();
In this example, integers are generated 10 times faster than they are consumed, leading to a bloating queue. This behavior exemplifies a failure to consider back-pressure.
By shifting from an eager approach to a lazy one, we can control data production:
function createStream(iterator) {
return new ReadableStream({
async pull(controller) {
const { value, done } = await iterator.next();
if (done) {
controller.close();
} else {
controller.enqueue(value);
}
},
});
}
This change ensures that data is consumed at a pace matching the generation. This is a more robust and proactive approach.
Managing Cancellation
In an infinite data stream, cancellation is crucial. Without proper management, memory leaks can occur.
We can correct this by employing a lazy approach with manual iteration:
function createStream(iterator) {
return new ReadableStream({
async pull(controller) {
const { value, done } = await iterator.next();
if (done) {
controller.close();
} else {
controller.enqueue(value);
}
},
});
}
This ties the generator's lifetime to the reader, canceling data production when reads cease.
Applying Stream Laziness to AI Interactions
Consider an AI service counting infinitely. If a user disconnects, without a proper handling mechanism, the server may continue requesting data, causing resource exhaustion.
A lazy approach ensures automatic resource management, promoting efficiency and stability.
Conclusion
The proper handling of back-pressure and cancellation in streams is fundamental for managing data flow effectively. By implementing a lazy approach, developers can maintain control over data production and consumption, thereby minimizing unnecessary resource usage.
The lessons learned here can be instrumental in developing more robust applications, particularly when dealing with vast amounts of data in AI integrations. Emphasizing efficiency and responsiveness aligns applications closer to user needs, resulting in a more robust and user-friendly experience.
Subscribe or follow me on Twitter for more content like this!
Comments ()