Building a Slogan Generator with Vercel AI SDK: A Step-by-Step Guide for Beginners
Let's build a quick project to get a feel for using the Vercel AI SDK
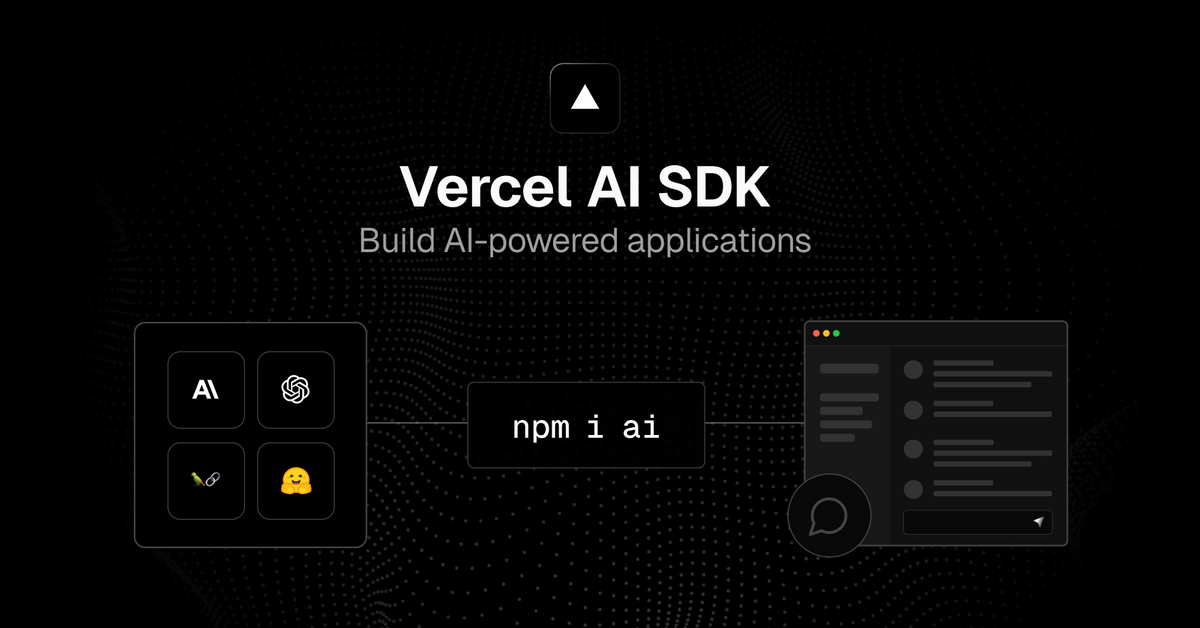
Building AI-powered user interfaces has never been easier thanks to the Vercel AI SDK. In this tutorial, you'll learn how to create a simple slogan generator application using the Vercel AI SDK. By the end of this guide, you'll be able to stream AI-generated content right into your user interface.
Subscribe or follow me on Twitter for more content like this!
This guide is tailored for beginners, so we'll be breaking down each step for clarity.
Prerequisites
Ensure you have:
- Node.js 18+ installed
- An OpenAI API key
Step 1: Create an Application
1.1 Create a New Next.js Application
pnpm dlx create-next-app my-ai-app
1.2 Navigate to the Directory
cd my-ai-app
Step 2: Install Dependencies
pnpm install ai openai
Step 3: Configure OpenAI API Key
3.1 Create .env.local File
touch .env.local
3.2 Edit .env.local File
OPENAI_API_KEY=xxxxxxxxx
Replace xxxxxxxxx with your actual OpenAI API key.
Step 4: Create an API Route
4.1 Create a File
mkdir -p app/api/completion && touch app/api/completion/route.ts
4.2 Edit the File
import OpenAI from 'openai';
import { OpenAIStream, StreamingTextResponse } from 'ai';
const openai = new OpenAI({
apiKey: process.env.OPENAI_API_KEY,
});
export const runtime = 'edge';
export async function POST(req: Request) {
const { prompt } = await req.json();
const response = await openai.completions.create({
model: 'text-davinci-003',
stream: true,
temperature: 0.6,
max_tokens: 300,
prompt: `Create three slogans for a business with unique features.
Business: ${prompt}
Slogans:`,
});
const stream = OpenAIStream(response);
return new StreamingTextResponse(stream);
}
Step 5: Wire up a UI
5.1 Create Client Component File
touch app/page.tsx
5.2 Edit the File
'use client'
import { useCompletion } from 'ai/react';
export default function SloganGenerator() {
const { completion, input, handleInputChange, handleSubmit } = useCompletion();
return (
<div className="mx-auto w-full max-w-md py-24 flex flex-col stretch">
<form onSubmit={handleSubmit}>
<input
className="fixed w-full max-w-md bottom-0 border border-gray-300 rounded mb-8 shadow-xl p-2"
value={input}
placeholder="Describe your business..."
onChange={handleInputChange}
/>
</form>
<div className="whitespace-pre-wrap my-6">{completion}</div>
</div>
);
}
Step 6: Running the Application
pnpm run dev
Conclusion
You've successfully built a slogan generator using Vercel AI SDK. Test it by entering a business description and see the AI-generated slogans.
Feel free to experiment and extend the functionality of this application further. Your imagination is the limit with AI, so explore the many possibilities that Vercel AI SDK offers.
Subscribe or follow me on Twitter for more content like this!
Comments ()