Swap Faces Seamlessly with the Faceswap Model
Seamlessly swap faces between pictures using AI!
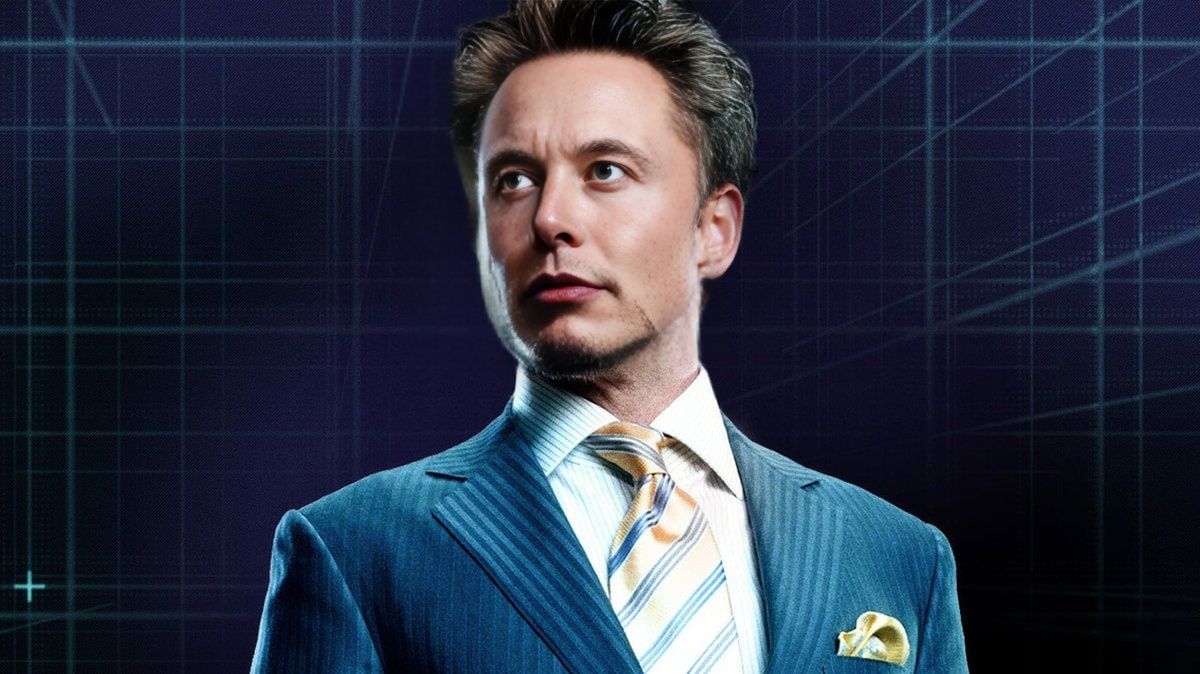
Have you ever imagined inserting yourself into iconic movie scenes or swapping the faces of your favorite actors? With the Faceswap model by lucataco, you can seamlessly replace faces in images to create entertaining effects and compelling visuals. This beginner-friendly guide will teach you how to harness the power of this pre-trained AI model to effortlessly swap faces.
The Faceswap model by lucataco enables seamlessly swapping faces between two images using deep learning. We'll use this pre-trained model to power our web app, built with some Node.js and the Replicate API. We'll also see how we can use AIModels.fyi to find similar models and decide which one we like.
- Accept two image URLs as input
- Swap the faces between the images with the Faceswap model
- Display the resulting face-swapped image
This will allow a user to easily swap faces from their browser! Ready to get started? Let's begin:
Subscribe or follow me on Twitter for more content like this!
Entertainment, Visual Effects, and Character Customization
The Faceswap model opens up creative possibilities in:
- Movies and shows: Swap actor faces seamlessly to replace characters or create surreal effects
- Games: Allow players to customize characters by swapping in their own faces
- Art projects: Combine faces in imaginative ways for unique creations
- Deepfakes: Generate photorealistic face swaps (use responsibly!)
This model empowers filmmakers, artists, gamers, and creators to redefine visual storytelling.
About Faceswap
Created by lucataco, the Faceswap model is an image-to-image model specifically designed for swapping faces between two individuals in a photo. It leverages deep learning techniques to detect and extract facial features. Once the features are extracted, it replaces one person's face with the other's, while maintaining the realistic nature of the image.
Technical Overview
Technically, the model utilizes convolutional neural networks to identify facial features and landmarks. Subsequent layers of the network are responsible for performing the actual swap, ensuring that lighting, orientation, and other factors align perfectly for a natural look.
Limitations
- The model may struggle with face orientations that are not front-facing.
- It may not perform well in poor lighting conditions.
- The quality of the face swap is dependent on the resolution of the input images.
Understanding the Inputs and Outputs of Faceswap
Before diving into the implementation, it's crucial to understand the data formats that Faceswap requires and provides.
Inputs
- target_image (file): This is the base image where the face will be placed.
- swap_image (file): This image provides the face that will be swapped onto the target image.
Outputs
- Output (URI string): The model returns a URI string where the swapped image can be accessed.
Now that we understand how the model works and what it can do, let's build our app!
Building our Face Swap App using Replicate's Face Swap Model
1. Installing Required Libraries
You'll need the following libraries to get started:
replicate
: To interact with the AI model hosted on Replicate.express
: A web framework for Node.js.
Install them with npm:
npm install replicate express
2. Authenticating with Replicate
Store your Replicate API token as an environment variable in your .env
file:
REPLICATE_API_TOKEN=your_token_here
In your code, you can then read this token:
const REPLICATE_API_TOKEN = process.env['REPLICATE_API_TOKEN']; // Use your token
3. Initializing the Replicate Client
Initialize the Replicate client for interacting with the services:
const Replicate = require('replicate');
const replicate = new Replicate({
auth: REPLICATE_API_TOKEN,
});
4. Creating a Function to Interact with the Model
This function will make a request to the face swap model:
async function getSwappedImage() {
try {
const output = await replicate.run(
"your_namespace/faceswap_model:version_hash",
{
input: {
image1: "path_or_url_to_first_image",
image2: "path_or_url_to_second_image",
}
}
);
console.log("Output from Replicate:", output);
return output;
} catch (error) {
console.error("Error running the model:", error);
}
}
5. Setting Up the Express Server
Set up an Express server to respond to GET requests:
const express = require('express');
const app = express();
const PORT = 3000;
app.get('/', async (req, res) => {
const swappedImageURI = await getSwappedImage();
res.send(`
<h1>Your Swapped Face</h1>
<img src="${swappedImageURI}" alt="Swapped Face" />
`);
});
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
6. Running the Application
Save this code into a file (e.g., app.js
). Make sure you have Node.js installed, and then run:
node app.js
Visit http://localhost:3000
to see the face-swapped image.
Full Code
Here's the entire code for your convenience:
require('dotenv').config();
const Replicate = require('replicate');
const express = require('express');
const app = express();
const PORT = 3000;
const REPLICATE_API_TOKEN = process.env['REPLICATE_API_TOKEN']; // Use your token
const replicate = new Replicate({
auth: REPLICATE_API_TOKEN,
});
async function getSwappedImage() {
try {
const output = await replicate.run(
"your_namespace/faceswap_model:version_hash",
{
input: {
image1: "path_or_url_to_first_image",
image2: "path_or_url_to_second_image",
}
}
);
console.log("Output from Replicate:", output);
return output;
} catch (error) {
console.error("Error running the model:", error);
}
}
app.get('/', async (req, res) => {
const swappedImageURI = await getSwappedImage();
res.send(`
<h1>Your Swapped Face</h1>
<img src="${swappedImageURI}" alt="Swapped Face" />
`);
});
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
Make sure to replace placeholders like your_namespace/faceswap_model:version_hash
and image paths with actual values relevant to your use case.
Taking It Further
Building a basic application for face-swapping is just the starting point. There are various avenues for improvement and additional features that could elevate the utility and user experience of your application. Here are five recommendations to take your faceswap app to the next level:
Real-Time Face Swap Capability:
- Allow users to upload a short video clip and implement real-time face swapping.
- Utilize libraries like OpenCV to handle real-time video processing.
Integrated Payments
- Monetize your application by charging users for additional features or for high-resolution face swap outputs.
- Integrate with popular payment gateways like Stripe using the stripe npm package.
Explore Different Models
- Your application can benefit from the multitude of AI models available on AIModels.fyi.
- Integrate models that offer unique face swap styles, or combine face swap with other effects like age progression or stylization.
Enhance User Experience (UX):
- Your current implementation is minimalistic. Improve it by:
- Introducing a modern UI using front-end frameworks like React or Angular.
- Adding a loading spinner to indicate model processing.
- Providing a gallery of face swap transformations for users to browse and compare.
User Profiles and History:
- Implement user authentication and profiles to allow users to save and review their past face swap projects.
- Use databases like MongoDB or PostgreSQL to persist user data.
Summary
In this post, we explored building a basic Node.js app for face-swapping using the Replicate library. We focused on:
- Setting up the necessary Node.js libraries, like Replicate and Express.
- Authenticating with Replicate to access the AI model.
- Implementing the face swap logic and displaying the swapped face via an Express server.
By leveraging the faceswap model hosted on Replicate, we could effortlessly create an app that swaps faces in images without building our AI model from scratch.
Conclusion
This tutorial showcased how Node.js and Replicate offer a flexible and efficient way to integrate sophisticated AI models like face swap into your application. The potential to enhance the app is enormous — from real-time face-swapping to adding monetization features. Replicate's ecosystem of AI models offers a wide range of opportunities for creativity and innovation.
This hands-on guide aims to demonstrate how developers can quickly build AI-powered applications, capitalizing on state-of-the-art generative capabilities through pre-trained models.
The expanding world of AI is a playground for developers, and I hope this post inspires you to build your own innovative apps enhanced by AI.
Thank you for reading. If you found this guide valuable, subscribe or follow me on Twitter for more content like this!
Subscribe or follow me on Twitter for more content like this!
Comments ()