LangChain Chat Models: An Overview
Taking a detailed look at LangChain's chat models
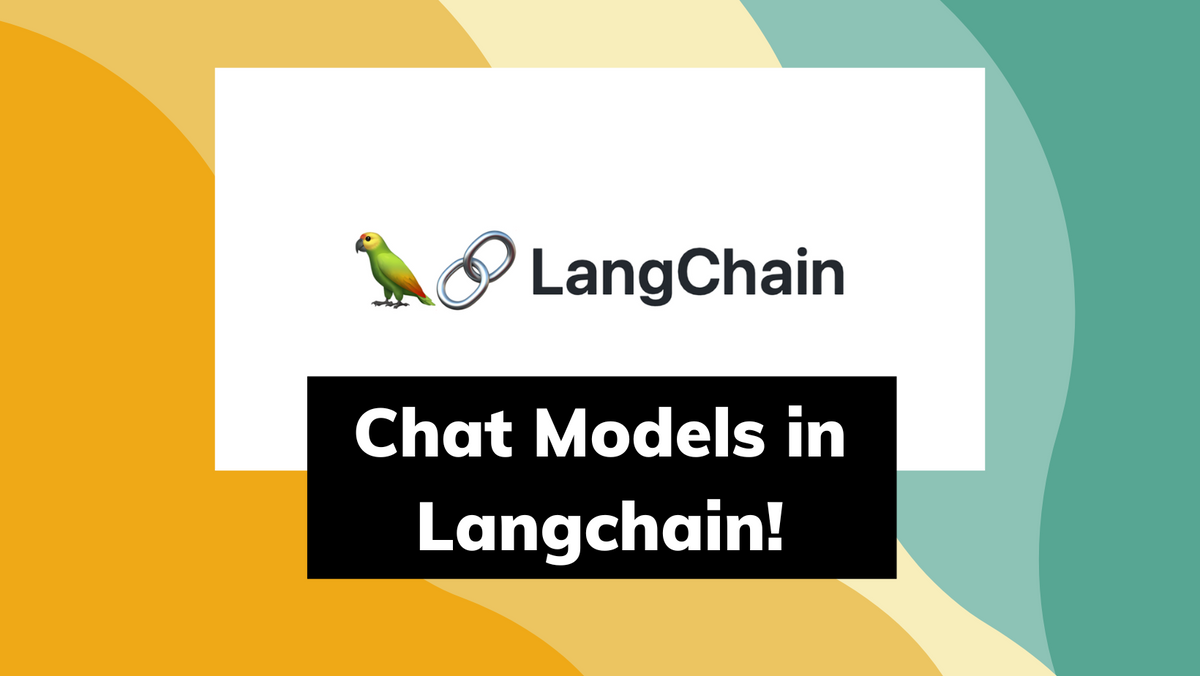
Hello there, AI enthusiasts! Today, we're going to have a closer look at LangChain Chat Models. LangChain is a fantastic tool that provides a standard interface for interacting with a variety of language models, including both text-based Large Language Models (LLMs) and Chat Models.
The Concept of Models in LangChain
Models are an essential part of LangChain. Rather than being a provider of models, LangChain serves as an interface allowing you to interact with diverse language models from other places. Currently, LangChain supports LLMs, Chat Models, and Text Embedding models.
The primary distinction between LLMs and Chat Models lies in their input and output structure. LLMs operate on a text-based input and output, while Chat Models follow a message-based input and output system.
It's important to note that chat model APIs are a relatively new feature, and they're still determining the most effective abstractions.
Getting Started with Chat Models
Chat Models are a variation of language models. While they use language models underneath, the interface they expose is somewhat different. Instead of a "text in, text out" API, they expose an interface where "chat messages" are the inputs and outputs.
At LangChain, they refer to a ChatMessage as the modular unit of information for a chat model. This usually includes a "text" field, signifying the content of the chat message. There are currently four different classes of ChatMessage supported:
HumanChatMessage
: A chat message sent from a human's perspective.AIChatMessage
: A message sent from the point of view of the AI system the human is communicating with.SystemChatMessage
: A message that provides the AI system with some information about the conversation. Typically sent at the start of a conversation.ChatMessage
: A generic chat message, not only with a "text" field but also an arbitrary "role" field.
LangChain presently supports the ChatOpenAI model (with gpt-4 and gpt-3.5-turbo), but they anticipate adding more in the future. To get started, all you need to do is use the call method of an LLM implementation, passing in a string input. Here's a simple example of how to initiate a conversation using the ChatOpenAI implementation:
import { ChatOpenAI } from "langchain/chat_models/openai";
import { HumanChatMessage } from "langchain/schema";
export const run = async () => {
const chat = new ChatOpenAI();
const response = await chat.call([
new HumanChatMessage(
"What is a good name for a company that makes colorful socks?"
),
]);
console.log(response);
// AIChatMessage { text: '\n\nRainbow Sox Co.' }
};
To get a deeper understanding, you can refer to the API references: ChatOpenAI and HumanChatMessage.
Integrations: Chat Models
LangChain offers several Chat Models implementations that integrate with various model providers. These include ChatOpenAI
, Azure ChatOpenAI
, and ChatAnthropic
. Each of these providers has a different way of getting instantiated. For example, to instantiate ChatOpenAI
:
import { ChatOpenAI } from "langchain/chat_models/openai";
const model = new ChatOpenAI({
temperature: 0.9,
apiKey: "your_openai_api_key"
});
The temperature
is a hyperparameter that adjusts the randomness of the model's responses. Higher values result in more diverse outputs, while lower values make the output more deterministic.
The apiKey
is your personal OpenAI API key, which authorizes you to use the model.
Similarly, you can instantiate Azure ChatOpenAI
and ChatAnthropic
:
// Azure ChatOpenAI
import { AzureChatOpenAI } from "langchain/chat_models/azure";
const model = new AzureChatOpenAI({
temperature: 0.9,
azureApiKey: "your_azure_api_key"
});
// ChatAnthropic
import { ChatAnthropic } from "langchain/chat_models/anthropic";
const model = new ChatAnthropic({
temperature: 0.9,
anthropicApiKey: "your_anthropic_api_key"
});
Note: Make sure to replace "your_openai_api_key"
, "your_azure_api_key"
, and "your_anthropic_api_key"
with your actual API keys.
Keep in mind that each provider may have specific parameters unique to their implementation. Always refer to the LangChain documentation or the provider's documentation for a comprehensive understanding of the parameters.
Conclusion
LangChain's Chat Models provide a unified approach to interacting with various AI language models. This versatility allows developers to switch between different providers seamlessly. While we've covered the basics of instantiating chat models from OpenAI, Azure, and Anthropic, there's a wealth of other functionality and customization available in LangChain. As an open-source project, LangChain encourages community contributions to drive its growth and evolution. With LangChain, the potential to create powerful language processing applications is at your fingertips.
Subscribe or follow me on Twitter for more content like this!
FAQs
What is LangChain?
- LangChain is an open-source project aimed at simplifying the use of various language models by providing a uniform interface. It supports several popular AI language model providers like OpenAI, Azure, and Anthropic.
How can I contribute to LangChain?
- As an open-source project, LangChain welcomes community contributions. You can contribute in many ways, such as adding new features, fixing bugs, improving the documentation, and more. Please refer to the project's contribution guidelines for more information.
What is the temperature
parameter in the instantiation code?
- The
temperature
parameter controls the randomness of the model's responses. Higher values produce more random outputs, while lower values make the model's outputs more deterministic.
What is an API key, and why do I need it?
- An API key is a code that identifies the calling program. It's used to track and control how the API is being used. For LangChain, you need to provide your provider-specific API key to authenticate your requests.
Where can I get my API key?
- You can obtain your API key from the respective provider's platform. For instance, for OpenAI, you can generate your API key from your OpenAI account's dashboard.
Is LangChain free to use?
- While LangChain is an open-source project and free to use, the underlying AI providers may charge for their services. Please check the specific pricing details of the provider you're planning to use.
Comments ()